Streamline Your Workflow with Automated Bitly URL Shortening
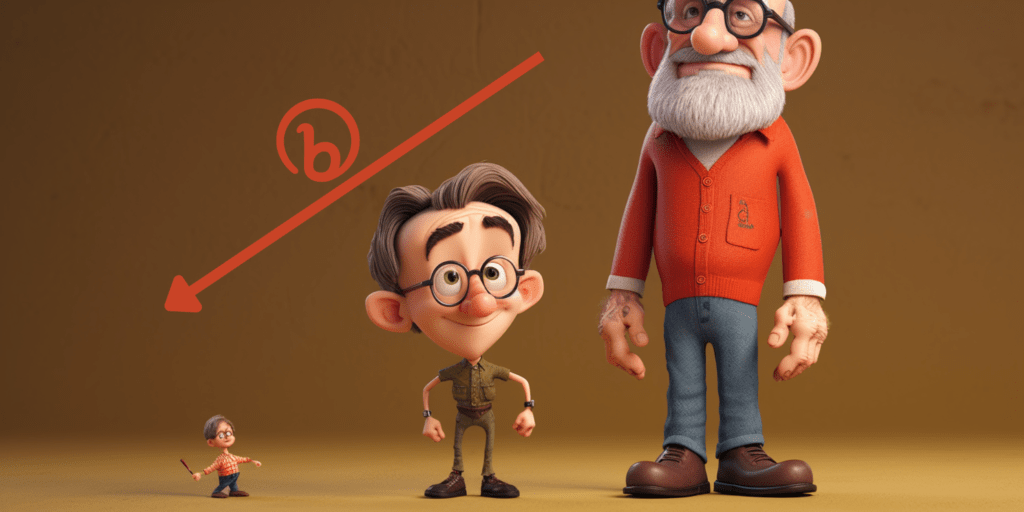
Introduction
Bitly is a popular link management platform that enables users to shorten, track, and optimize URLs. With its powerful features, Bitly has become an indispensable tool for marketers, content creators, and developers alike. In this blog post, we’ll walk you through the process of automating URL shortening using the Bitly API and Python. We’ll cover everything from setting up your environment to obtaining an API key, and finally, writing and running the Python script.
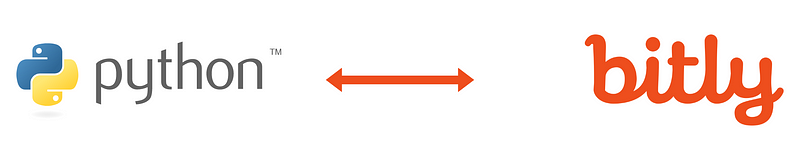
What is Bitly?
Bitly is a URL-shortening service and a link management platform that offers users the ability to turn long, cumbersome URLs into short, easy-to-remember links. With Bitly, you can also track link performance, analyze user engagement, and optimize your marketing campaigns. Shortened URLs are particularly useful when sharing links on social media, in emails, or in any situation where space is limited.
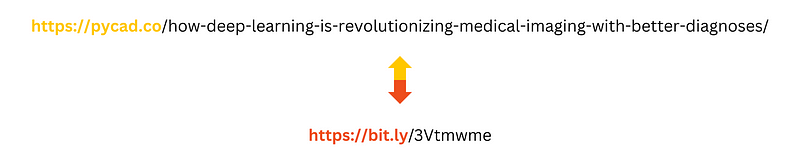
Setting up your environment
Before you begin, it’s essential to set up a proper environment to ensure a smooth and hassle-free experience. We recommend creating a Conda environment with the necessary dependencies installed. If you don’t have Conda installed on your system, you can download it from the official website: https://docs.conda.io/en/latest/
Follow these steps to create a new Conda environment:
Update Conda:
conda update conda
Create a new environment with Python 3.9 and required packages:
conda create -n myenv python=3.9 requests
Activate the new environment:
conda activate myenv
Install OpenSSL:
conda install openssl
Additionally, you may need to install OpenSSL on your system if it’s not already installed. You can find instructions for your specific operating system here: https://www.openssl.org/source/
Obtaining a Bitly API Key
To use the Bitly API, you’ll need an API key. Follow these steps to obtain one:
a. Sign up for a Bitly account: https://bitly.com/
b. Log in to your account and navigate to your account settings.
c. Find the API section and generate an access token.
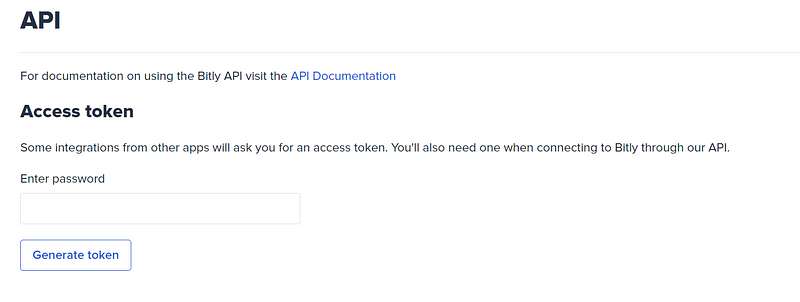
Automating URL Shortening with Python
Now that your environment is set up and you have your Bitly API key, let’s dive into the Python script.
import requests
def shorten_url(api_key, long_url):
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json',
}
data = {
"long_url": long_url,
}
response = requests.post('https://api-ssl.bitly.com/v4/shorten', json=data, headers=headers)
if response.status_code == 200:
return response.json()['link']
else:
raise Exception(f"Error shortening URL: {response.status_code}, {response.text}")
if __name__ == "__main__":
api_key = 'your_bitly_api_key_here'
long_url = 'https://www.example.com/very-long-url'
try:
short_url = shorten_url(api_key, long_url)
print(f'Shortened URL: {short_url}')
except Exception as e:
print(e)
Make sure to replace your_bitly_api_key_here
with your actual Bitly API key.
How Dropbox Automations works (Plan)
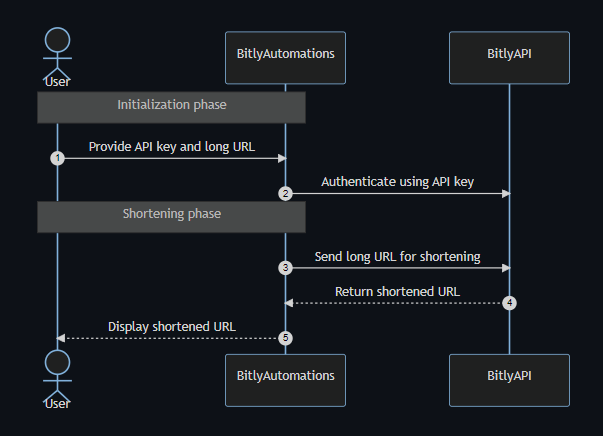
Conclusion
In this blog post, we’ve demonstrated how to automate Bitly URL shortening using Python and the Bitly API. By streamlining this process, you can save time and boost productivity in your marketing campaigns, social media sharing, and other digital efforts. By following the steps outlined in this post, you can set up your Conda environment, obtain your Bitly API key, and create a simple Python script to shorten URLs automatically. This technique opens up new possibilities for integrating Bitly functionality into your projects and applications, making your workflow more efficient and effective. Happy coding!
Link to the code in GitHub:
https://github.com/amine0110/bitly-automation

Don’t miss out on the latest news and trends in medical imaging. Subscribe to our newsletter at pycad.co/join-us to stay informed.
join-us – PYCAD
Computer Vision Newsletter Master the Field of Medical Imaging with Python Previously Sent Newsletters March 6, 2023…pycad.co