If you want to draw something on an image or a canvas in Tkinter. There are some steps that you should do first. Follow me in this blog step by step to see how you can easily do it.
If you have already understand how does it work, then you can find the final code here.
Amine
The steps
- Create the application
- Create a canvas
- Put an image on the canvas
- Draw lines on the canvas using the mouse
Create the application
To create the application, we will use only 4 lines of code (if you have never used Tkinter before you can check my other blog about how to create a Tkinter application for beginners at this link). However, I will give you the 4 lines of code to create an empty application that we need in this project.
from tkinter import *
app = Tk()
app.geometry("400x400")
app.mainloop()
The first line is to include the Tkinter library. The second line is to create the application. The third is to change the application’s dimensions and finally the last line is to pour the application as the main loop so that it will stay running.
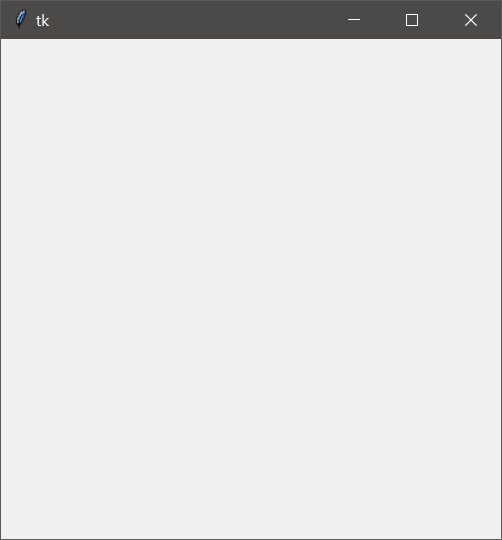
Create a canvas in Tkinter
Now after that, we have created the empty application. We need to put a canvas on it so that we can draw on it. To create a canvas it is very is, we will use the Canvas function of Tkinter. You can see how to do it in these two lines of code.
canvas = Canvas(app, bg='black')
canvas.pack(anchor='nw', fill='both', expand=1)
In the first line, I have created the canvas with a black background color. Then in the second line, I packed it in the application but you can see that there are some other parameters that I will talk about now.
There is the anchor is to choose the starting point of the canvas. For that, I put ‘nw’ for nor-west which means the first point of the canvas will be at the same starting point of the widget which is (0, 0).
And for the fill and expand parameters are to stretch the canvas on the whole area of the application so that we can use the whole size. And even if we change the size of the window, the canvas size will change automatically.
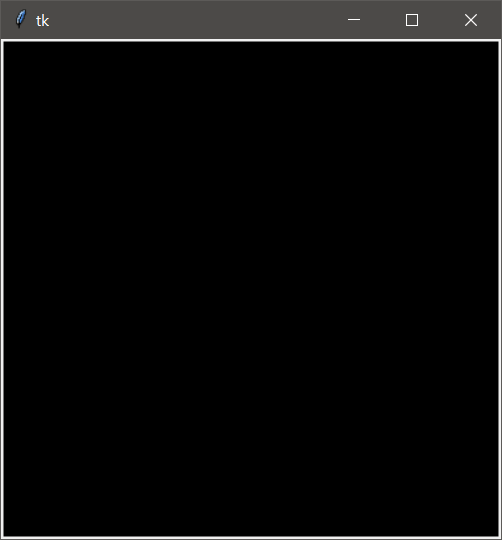
How to put an image in a Canvas
Now it comes the step to put an image in the canvas, for that I’m using pillow library to open the image, resize it and make it ready for a Tkinter application.
Then you should create a canvas image using the function ‘create_image’ so that you you can draw on it.
Here is the lines of code to do that:
image = Image.open("image.jpg")
image = image.resize((400,400), Image.ANTIALIAS)
image = ImageTk.PhotoImage(image)
canvas.create_image(0,0, image=image, anchor='nw')
You can see that I did a resizing to the image so that it fits with the canvas (you can check the video, it has more details about that).
Here is the image on the application:
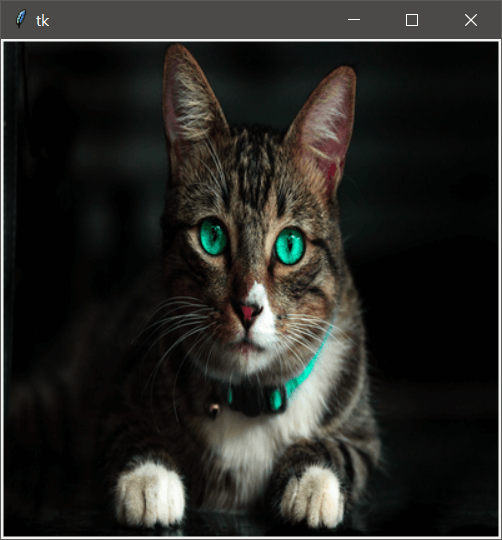
Draw on the image using the mouse
Now comes the final step, after putting the image on the canvas, we need to create two functions for the drawing. The first one is to get the x and y of the first click with the mouse button then the second function will take the coordinate of the previous click with the new click and draw a line. Because to draw a line we need only 2 points, the starting one and the ending one. For that, if we stay clicking at the button and we drag to draw means that we are creating a lot of point with a very small distance between. Using these points we draw lines so that it will be something continuous.
Here are the two functions that you need to do what I was saying:
def get_x_and_y(event):
global lasx, lasy
lasx, lasy = event.x, event.y
def draw_smth(event):
global lasx, lasy
canvas.create_line((lasx, lasy, event.x, event.y),
fill='red',
width=2)
lasx, lasy = event.x, event.y
Now as any normal program, if we declare a function it does nothing if we don’t call it. The same thing here, we declared these two functions but we need to call them. So the right time to call theme is when we click on the image.
For that you need to add these to lines to do the call of that functions:
canvas.bind("<Button-1>", get_x_and_y)
canvas.bind("<B1-Motion>", draw_smth)
The word button 1 is to say that when we click on the first mouse button then it will call the functions. Otherwise, if you want to call these functions (start drawing) only when after clicking on a button. In this case, all you need to do is to put these two last lines in the callback function of the button.
Here is the final result:
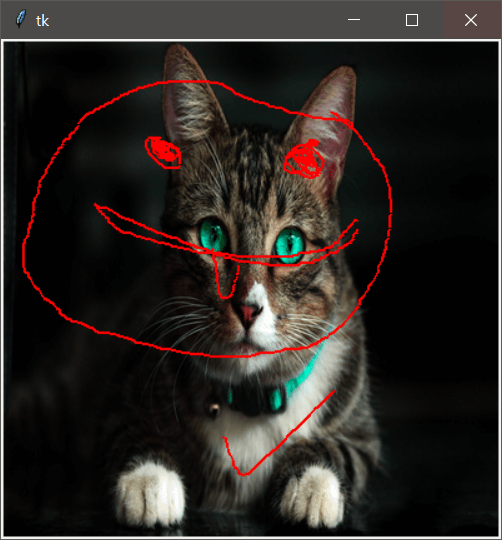
Now we are at the end of this blog I hope that you like it, if you want to use the whole program then you can find it in this link.
Happy learning
If you want more about Tkinter. You can find my course for beginners at this link with a huge discount, only for you.
Find a sponsor for your web site. Get paid for your great content. shareasale.com.