Tools: Python, Pydicom
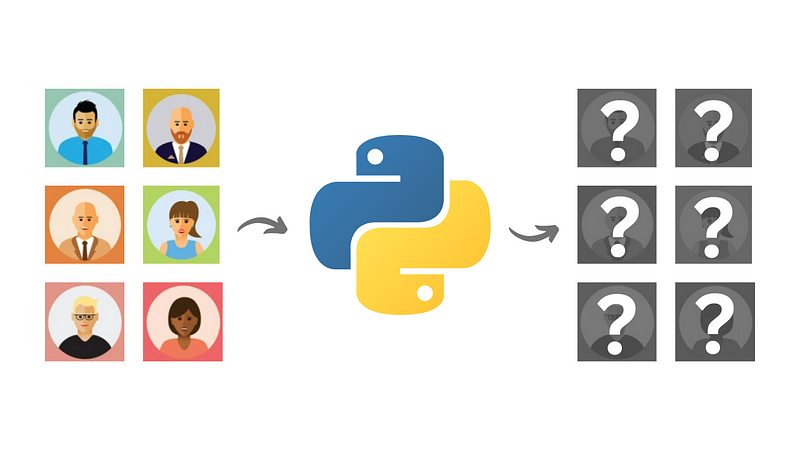
Task
In this blog post, I will show you how you can anonymize Dicom files so that you can hide the identity of the patients.
Packages to Install
pip install pydicom
pip install glob2
If you want to know more about this library, then you can check their documentation here.
The code
The idea behind this operation is to use Pydicom to load the Dicom file and then change the variable Dicom file. You can turn PatientName
into anything you want: a random name, anonymous (the convention), or even an empty string.
import pydicom as pm
dicom_path = 'path/to/dicom/file'
dicom_file = pm.dcmread(dicom_path)
print('The patient name is: ', dicom_file.PatientName)
Here we print the actual patient name: (I put my name on it because it was already anonymized)
The patient name is: Mohammed El Amine Mokhtari
To do the Dicom anonymization:
output_path_dicom = 'anonymous_slice.dcm'
dicom_file.PatientName = 'Anonymous' # or 'Amine', or ' ', ...
dicom_file.save_as(output_path_dicom)
Now you have an anonymized slice, if you want to check then you can do the same thing, upload the Dicom file and print the PatientName
variable.
Anonymize a Group of Dicom Files
The Dicom anonymization should be performed on all of the case’s slices; here is the code to do so.
import pydicom as pm
from glob import glob
import os
def anonymize_dicom(in_path, out_path, PatientName='Anonymous'):
dicom_file = pm.dcmread(in_path)
dicom_file.PatientName = PatientName
dicom_file.save_as(out_path)
if __name__ == '__main__':
path_to_dicoms = 'path/to/all/the/dicoms'
slices = glob(os.path.join(path_to_dicoms, '*'))
for slice_ in slices:
anonymize_dicom(slice_, slice_)
I put the same path for the in_path
and the out_path
so that we overwrite the same file, but if you prefer keeping the Dicoms having the exact names and creating new anonymized Dicoms, then you can give another out_path
. For the PatientName argument, I kept it empty because by default it will be ‘Anonymous’ but if you want something else then you can add it to the arguments.
Note
In this blog post, I spoke only about changing the name of the patient, but you can change even other information such as StudyID
, StudyData
, PatientBirthDate
, and so on.
dicom_file.PatientBirthDate = 'Something'
dicom_file.StudyID = 'Something'
dicom_file.StudyDate = 'Something'
This concludes this blog post; I hope you found it useful. If so, don’t forget to keep a reaction because it will help me do more 🙂
Related articles
- The best libraries for medical imaging and Python.
- Convert nifti to stl.
- Visualize and annotate Dicom files.
- Convert JPG/PNG into Dicom files.
- Convert a Dicom file into JPG/PNG.
🆕 NEW
Learn how to effectively manage and process DICOM files in Python with our comprehensive course, designed to equip you with the skills and knowledge you need to succeed.